27 Sep 2011 in
ASP.NET MVC
This subject seems to be really basic when developing a web application but it seems that it’s very discussed on the web too. In a lot of forum threads or blog posts we can find very various methods to handle HTTP exception within an ASP.NET MVC application. This post exposes a way to do that. I’m not pretending that it’s the best way but I think that it’s an elegant one
(so don’t hesitate to comment on this post if you’ve remarks or suggestions).
What’s the real need ?
I think that developers should manage HttpException in an other way than other exceptions for 3 main reasons :
- Respect of web-standards (a not found error returns a 404 http code, that’s it !)
- SEO : search engines use http codes (because it’s standards) to build their indexes.
- The user : a user-friendly page is already better than a stack trace…
I think that the most important is that the error code is well returned by the server : for example the URL http://www.mywebsite.com/Pages/some-page-that-does-not-exists is called the server should return a 404 status code and not a 302 (found) followed by a 200 (or 404) from a specific error page like http://www.mywebsite.com/Errors/Error404).
When HttpException are thrown in an ASP.NET MVC application ?
HttpException are thrown by the ASP.NET MVC engine or by the application code, directly in the asp.net controllers of your apps :
throw new HttpException(404, "Not found");
In some cases, they can also be thrown by the server (for internal errors, for example). But all HttpException can be handled in the application.
How to handle these exceptions ?
After a lot of researches and some tests I think that http exceptions should be handled in two places :
- In a base controller inherited by all the controllers of the application
- In the Global.asax file
Handle http exception in the controller :
Exceptions that are thrown by the developer directly in the application code can be handle in a base controller. For example :
public ActionResult Product(int id)
{
var product = _unitOfWork.GetProduct(id);
if(product == null)
throw new HttpException(404, "Le produit est introuvable");
return View(product);
}
By inheriting the System.Web.Mvc.Controller class it’s possible to override an OnException
method. This method is called when an exception occurs in a controller method (action) :
protected override void OnException(ExceptionContext filterContext) {
base.OnException(filterContext);
if (filterContext.Exception != null) {
filterContext.ExceptionHandled = true;
if (filterContext.Exception is HttpException) {
if (!ControllerContext.RouteData.Values.ContainsKey("error")) {
ControllerContext.RouteData.Values.Add("error", filterContext.Exception);
}
var httpException = (HttpException) filterContext.Exception;
switch (httpException.GetHttpCode()) {
case 404:
filterContext.HttpContext.Response.StatusCode = 404;
filterContext.HttpContext.Response.StatusDescription = httpException.Message;
View("Error404", null).ExecuteResult(ControllerContext);
break;
case 500:
filterContext.HttpContext.Response.StatusCode = 500;
filterContext.HttpContext.Response.StatusDescription = httpException.Message;
View("Error500", null).ExecuteResult(ControllerContext);
break;
default:
filterContext.HttpContext.Response.StatusDescription = httpException.Message;
View("GenericError", null).ExecuteResult(ControllerContext);
break;
}
}
//autre traitement si pas HttpException (log par exemple...)
}
}
As you can see in this code snippet the first step is to check that the exception is an HttpException. If yes, a redirection to an appropriate error page is done just after setting the status code and status description of the http response. The views are in the Shared
folder of the application.
Now http exceptions that are thrown in a controller return the good status code and redirect to a user friendly page.
Handle HTTP exceptions in the Global.asax :
It’s in the Global.asax file that all other http exception are handled. To to that you should subscribe to the Error event of the mvc application (in the Init method override) :
public override void Init()
{
base.Init();
this.Error += new EventHandler(MvcApplication_Error);
}
In the Error event handler, initialize an errors-dedicated controller and create appropriate route data to work with the error :
var routeData = new RouteData();
routeData.Values.Add("controller", "Errors");
var lastException = Server.GetLastError();
if (lastException is HttpException) {
var httpException = (HttpException) lastException;
switch(httpException.GetHttpCode()) {
case 404:
routeData.Values.Add("action", "Error404");
break;
case 500:
routeData.Values.Add("action", "Error500");
break;
default:
routeData.Values.Add("action", "GenericError");
break;
}
}
else
{
routeData.Values.Add("action", "GenericError");
}
routeData.Values.Add("exception", lastException);
Server.ClearError();
IController errorController = _unityContainer.Resolve<ErrorsController>();
errorController.Execute(new RequestContext(
new HttpContextWrapper(Context), routeData));
The ErrorsController :
public class ErrorsController : Controller
{
public ActionResult Error404() {
Response.StatusCode = 404;
Exception exception = null;
if(RouteData.Values.ContainsKey("exception")) {
exception = (Exception) RouteData.Values["exception"];
}
return View(exception);
}
public ActionResult Error500() {
Response.StatusCode = 500;
Exception exception = null;
if (RouteData.Values.ContainsKey("exception"))
{
exception = (Exception)RouteData.Values["exception"];
}
return View(exception);
}
public ActionResult GenericError() {
Exception exception = null;
if (RouteData.Values.ContainsKey("exception"))
{
exception = (Exception)RouteData.Values["exception"];
}
return View(exception);
}
}
As you can see each action defines itself the http status code of the http response.
Now all http exceptions are handled in a user and SEO friendly way in your ASP.NET MVC applications !
Hope this helps 
read more
27 Sep 2011 in
ASP.NET MVC
Bien que cela soit un sujet qui paraissent essentiel et surtout basic
lorsque l’on développe une application web (avec ASP.NET MVC ou pas d’ailleurs), ce sujet semble avoir fait couler beaucoup d’encre sur le web ! (si je puis dire…). En effet, il semble qu’il règne une sorte de flou
autour de ce sujet ou chacun utilise une méthode différente pour gérer les erreurs HTTP. Je ne sais pas si on peut parler ici de bonne pratique
mais en tout cas, ce post présente un moyen que je juge efficace et suffisament générique pour gérer les Http Exception dans une application ASP.NET MVC ! (n’hésitez pas à me faire par de vos remarques / suggestions en commentaire
)
Quel est le réel besoin ?
Au final, pourquoi souhaite-t-on traiter les erreurs HTTP de manière différente des autres erreurs ? A mon humble avis, pour 3 raisons principalement :
- Le respect des standards (une page qui n’existe pas c’est un 404, c’est tout !)
- La SEO : les moteurs de recherche utilisent tous les codes HTTP (comme c’est standard) pour construire leurs index.
- L’utilisateur : on souhaite en général personnaliser la page d’erreur sur laquel arrive l’utilisateur !
Ce qui importe donc, c’est avant tout que le code d’erreur soit bien renvoyé : si j’appelle une URL http://www.monsite.com/Pages/Page-qui-n-existe-pas
je souhaite que ce soit bien cette page là qui me retourne un code de status 404 et non pas un code 302 (trouvé) suivi d’un 200 (ou 404, à la limite, mais non) sur une URL de type http://www.monsite.com/Errors/Error404
.
Quand est-ce que des HttpException sont levées en ASP.NET MVC ?
Les exceptions sont levées soit par le moteur ASP.NET MVC, soit dans votre code :
throw new HttpException(404, "Not found");
Elles peuvent également être levées au niveau du serveur (erreur interne 500, par exemple), mais dans tous les cas, il est possible de les gérer !
Après pas mal de recherches et de tests, je pense qu’il y a deux endroits où il faut gérer les exceptions :
- Dans un contrôleur de base dérivé par tous les contrôleurs d’une application ASP.NET MVC
- Dans le fichier Global.asax
Gestion des erreurs dans un contrôleur de base :
Au sein du contrôleur, on gèrera les exceptions qui ont été levées intentionnellement par le développeur. Par exemple :
public ActionResult Product(int id)
{
var product = _unitOfWork.GetProduct(id);
if(product == null)
throw new HttpException(404, "Le produit est introuvable");
return View(product);
}
Lorsque l’on dérive la classe System.Web.Mvc.Controller, il est possible de surcharger une méthode OnException
: celle-ci est appelée lorsqu’une exception est levée dans le contrôleur.
protected override void OnException(ExceptionContext filterContext) {
base.OnException(filterContext);
if (filterContext.Exception != null) {
filterContext.ExceptionHandled = true;
if (filterContext.Exception is HttpException) {
if (!ControllerContext.RouteData.Values.ContainsKey("error")) {
ControllerContext.RouteData.Values.Add("error", filterContext.Exception);
}
var httpException = (HttpException) filterContext.Exception;
switch (httpException.GetHttpCode()) {
case 404:
filterContext.HttpContext.Response.StatusCode = 404;
filterContext.HttpContext.Response.StatusDescription = httpException.Message;
View("Error404", null).ExecuteResult(ControllerContext);
break;
case 500:
filterContext.HttpContext.Response.StatusCode = 500;
filterContext.HttpContext.Response.StatusDescription = httpException.Message;
View("Error500", null).ExecuteResult(ControllerContext);
break;
default:
filterContext.HttpContext.Response.StatusDescription = httpException.Message;
View("GenericError", null).ExecuteResult(ControllerContext);
break;
}
}
//autre traitement si pas HttpException (log par exemple...)
}
}
Comme vous pouvez le constater, ce code vérifie si l’exception est de type HttpException, si c’est le cas, elle redirige vers une vue d’erreur après avoir renseigner un status code et un status description au niveau de la réponse.
NB : ces vues sont placées dans le dossier Shared
des vues partagées.
Premier objectif atteind : une erreur HTTP levée dans un contrôleur se traduit par une page d’erreur user friendly
et une réponse HTTP SEO/Standards friendly
puisque retournant le bon code HTTP sur la bonne URL !
Gestion des erreurs HTTP dans le Global.asax :
Dans le Global.asax, on va gérer toutes les autres erreurs HTTP qui pourraient être levées dans l’application.
Pour se faire, on surcharge d’abord la méthode Init afin de s’abonner à l’événement Error :
public override void Init()
{
base.Init();
this.Error += new EventHandler(MvcApplication_Error);
}
Dans la méthode MvcApplication_Error, on récupère la dernière erreur du serveur, on vérifie si celle-ci est de type HttpException. Si oui, on instancie un contrôleur spécialisé dans la gestion des erreurs (ici ErrorsController, détaillé plus bas) on exécute le rendu de la vue en lui passant les bonnes RouteData :
var routeData = new RouteData();
routeData.Values.Add("controller", "Errors");
var lastException = Server.GetLastError();
if (lastException is HttpException) {
var httpException = (HttpException) lastException;
switch(httpException.GetHttpCode()) {
case 404:
routeData.Values.Add("action", "Error404");
break;
case 500:
routeData.Values.Add("action", "Error500");
break;
default:
routeData.Values.Add("action", "GenericError");
break;
}
}
else
{
routeData.Values.Add("action", "GenericError");
}
routeData.Values.Add("exception", lastException);
Server.ClearError();
IController errorController = _unityContainer.Resolve<ErrorsController>();
errorController.Execute(new RequestContext(
new HttpContextWrapper(Context), routeData));
Voilà le code du contrôleur ErrorsController :
public class ErrorsController : Controller
{
public ActionResult Error404() {
Response.StatusCode = 404;
Exception exception = null;
if(RouteData.Values.ContainsKey("exception")) {
exception = (Exception) RouteData.Values["exception"];
}
return View(exception);
}
public ActionResult Error500() {
Response.StatusCode = 500;
Exception exception = null;
if (RouteData.Values.ContainsKey("exception"))
{
exception = (Exception)RouteData.Values["exception"];
}
return View(exception);
}
public ActionResult GenericError() {
Exception exception = null;
if (RouteData.Values.ContainsKey("exception"))
{
exception = (Exception)RouteData.Values["exception"];
}
return View(exception);
}
}
NB : notez que c’est dans chaque action que l’on défini le status code de la réponse HTTP !
Je vous passe le code des vues qui n’a pas grand intérêt (mise à part que l’on peut réutiliser ici les vues du dossier Shared
évidemment)
Deuxième objectif atteind : une erreur HTTP levée autre part que dans un contrôleur se traduit par une page d’erreur user friendly
et une réponse HTTP SEO/Standards friendly
puisque retournant le bon code HTTP sur la bonne URL !
A bientôt ! 
read more
22 Sep 2011 in
Web Development
As you probably already know the last version of Entity Framework (4.1) supports a new development scenario called Code first
(also called code only in some cases). It consists to develop the .NET entities (POCOs) before the SQL relational model, create the database, map these entities to table and columns at runtime according the class definitions. To do that, there is no need of an EDMX file, metadata and mapping are based on conventions and attributes.
Entity Framework 4.1 provides a lot of new functionalities that may be used even if the code first
approach is not applied for many reasons (existing database, database created and maintained by a DBA that doesn’t know .NET or Entity Framework…). To be able to use the new features of Entity Framework 4.1 it’s possible to continue with the database first approach and use an EDMX file to do the mapping between .NET objects (POCOs) and SQL tables and columns. This post explains how to do that !
Import the Entity Framework 4.1 library
To use EF 4.1 it’s recommended to reference the related NuGet package using the NuGet management console in Visual Studio. Right click on the project that should reference the library and choose Manage NuGet packages…

In the NuGet management console, search for Entity Framework
and click Install to download and reference the EntityFramework.dll library.
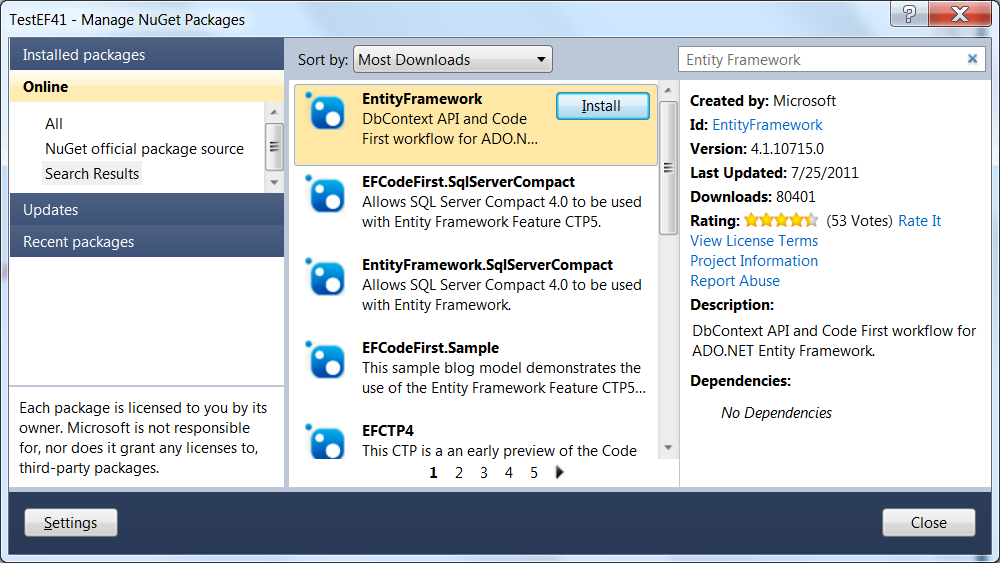
Download the T4 code generation templates for EF 4.1
To generate the DbContext and the .NET entities from the EDMX definition it’s possible to download two code generation templates from the Visual Studio Extensions manager. Open it and search for DbContext
in the online gallery :
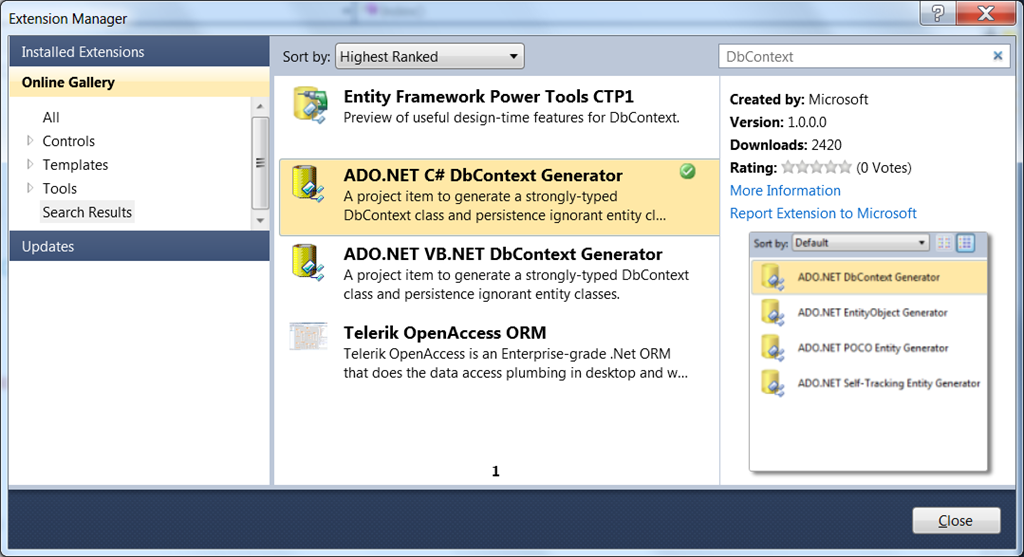
Install the ADO.NET C# DbContext Generator
item (or VB.NET).
When an ADO.NET entity data model is added to a project, it uses the default code generation template. To avoid this, you have to remove this tool in the property window of the EDMX file :
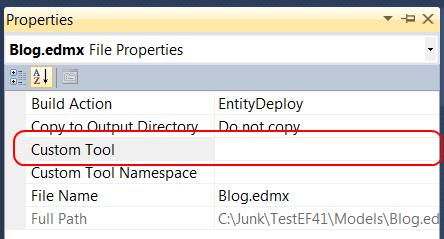
Be careful if you’re working on an existing project where generated code may be in use !
Add the templates to the project
Now you’ve to add the two generation code templates to the project. To do that add a new item of type ADO.NET C# DbContext Generator
from the add item menu :
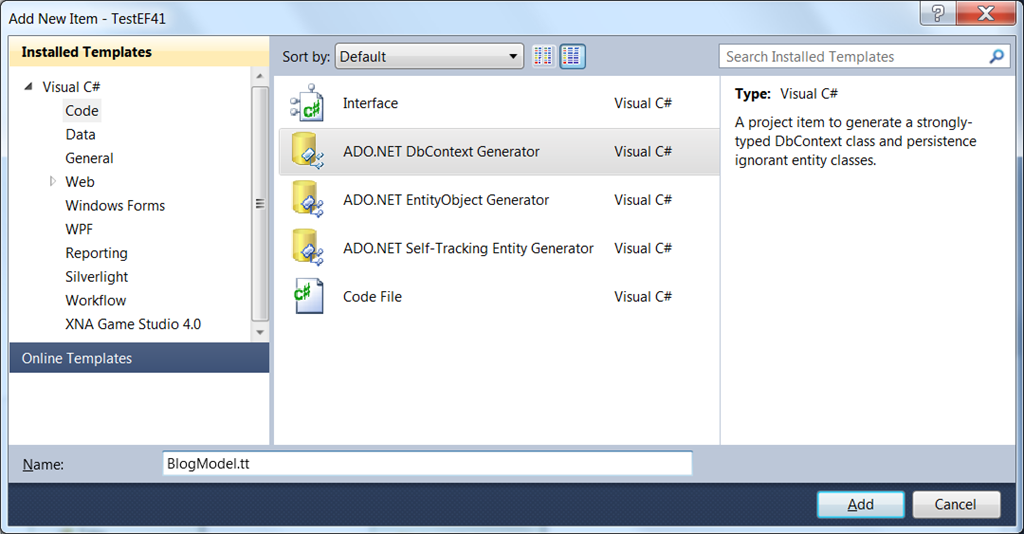
In these two files, find the text $edmxInputFile and replace it by the relative path to the EDMX file.
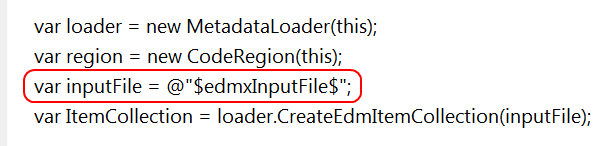
Save the files. Automatically the DbContext and the entities are generated :
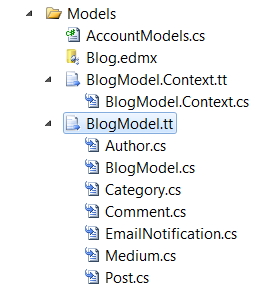
Now you are ready to use the DbContext and query the database through LINQ to Entites !
using (var dbContext = new BlogContainer()) {
var posts = dbContext.Posts.Where(p => p.IsPublished).ToList();
return View(posts);
}
After each change on the edmx file just run the custom tool on the two T4 templates to regenerate the DbContext and the entities.
Hope this helps 
read more
19 Sep 2011 in
Web Development
Le titre de ce post vous a peut-être suppris puisque l’une des nouveautés la plus plébicitée de Entity Framework 4.1 est la possibilité de s’affranchir d’un modèle EDMX et d’adopter une stratégie Code First : les entités .NET permettent de générer le modèle de données SQL.
Pourtant, Entity Framework 4.1 apporte de nombreuses autres nouveautés, notamment en terme de gestion de l’état des entités, la mise à jour d’entités dans un contexte state-less (notamment), de requêtage… via des méthodes beaucoup plus simples que précédement.
Ce post à pour but d’expliquer comment utiliser Entity Framework 4.1 tout en continuant d’utiliser un EDMX et une stratégie Database First !
Importer la librairie Entity Framework 4.1
Pour pouvoir utiliser EF 4.1, le plus simple est de référencer le package NuGet associé. Faites un clic droit sur votre projet Visual Studio puis cliqué sur Manage NuGet packages…
:

Dans la fenêtre qui s’affiche, recherchez Entity Framework :
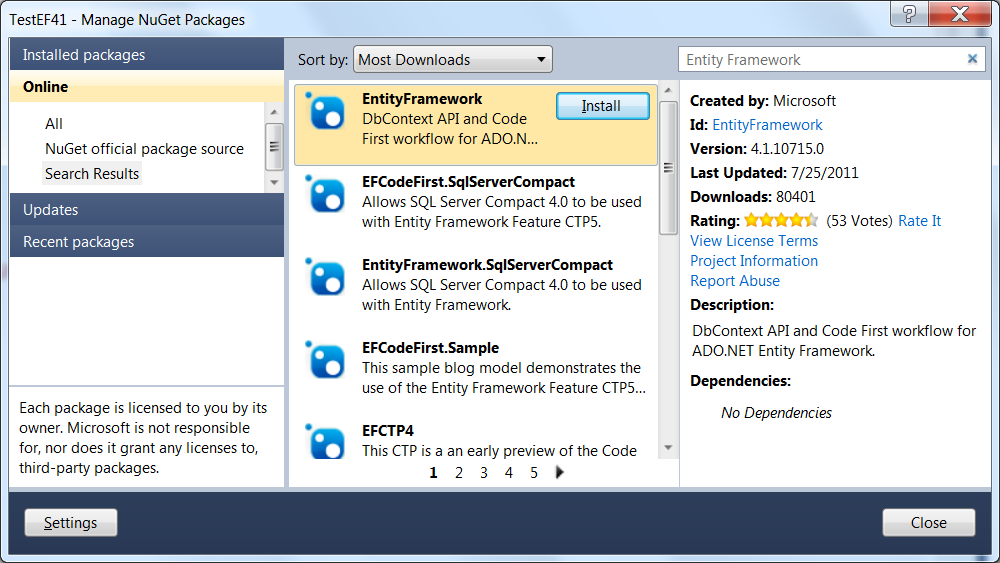
Cliquez sur Install
, acceptez les conditions. La librairie EntityFramework.dll va être téléchargée et référencée dans le projet.
Télécharger les templates de génération de code (T4) pour EF 4.1
Afin de générer le code propre à EF 4.1 (entités et DbContext) il est possible de télécharger un template d’item via le gestionnaire d’extension de Visual Studio. Placez-vous dans la galerie en ligne et recherchez DbContext :
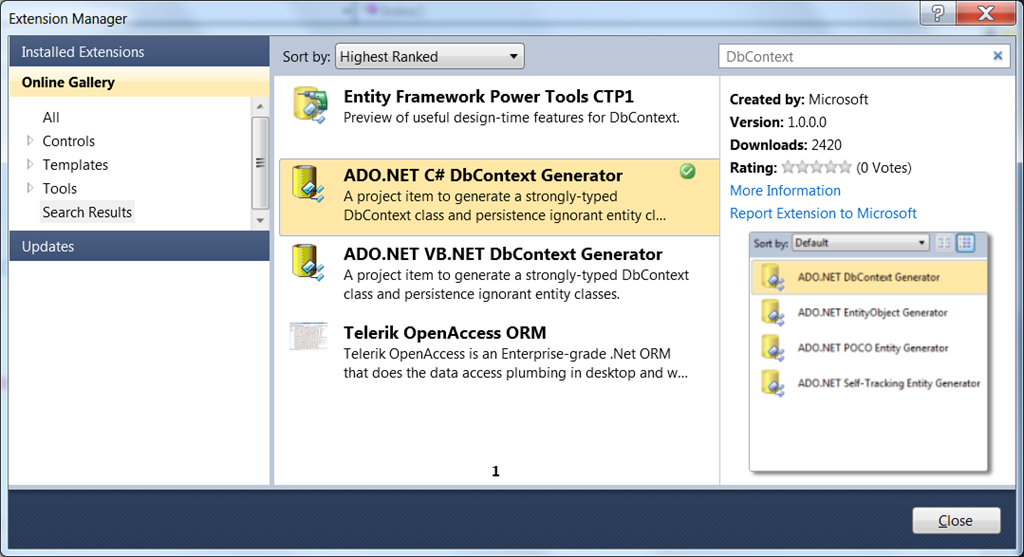
Installez l’élément ADO.NET C# DbContext Generator
(ou VB si vous le souhaitez
).
Supprimer l’outil de génération de code de votre EDMX
Afin que le code ne soit plus généré par l’EDMX, éditez les propriétés de ce dernier et supprimer l’outil personnalisé :
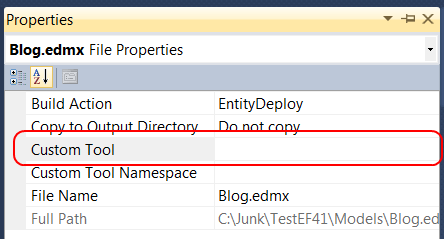
Attention : ne faites pas celà dans un projet qui utilise les éléments de code généré par l’EDMX Entity Framework classique
.
Ajouter les templates au projet
Maintenant, ajoutez un nouvel item de type ADO.NET C# DbContext Generator
au projet.
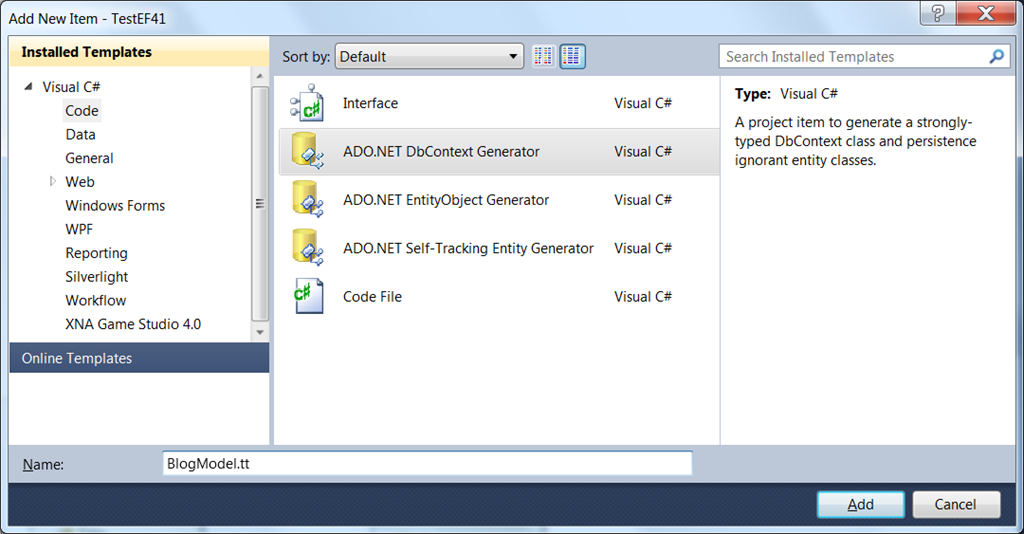
Identifiez la ligne de code suivante dans chacun des templates et modifiez la chaine $edmxInputFile$ par le chemin relatif vers votre fichier EDMX.
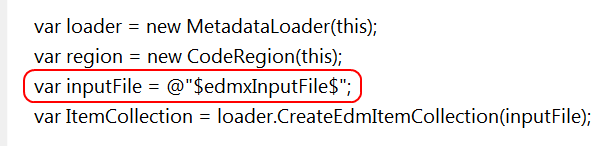
Dès lors, lorsque vous enregistrez les templates, du code devrait être généré : les entités et le DbContext.
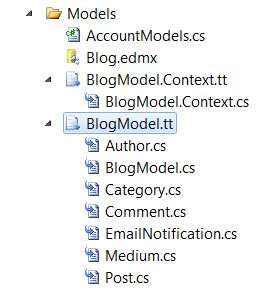
Et voilà, vous pouvez utilisez le contexte généré pour attaquer votre base de données !
using (var dbContext = new BlogContainer()) {
var posts = dbContext.Posts.Where(p => p.IsPublished).ToList();
return View(posts);
}
Après modification de l’EDMX, il suffit de regénérer les templates T4 pour les mettre à jour et de recompiler le tout 
A bientôt 
read more
13 Sep 2011 in
ASP.NET MVC
Since ASP.NET 4 was released it’s possible to write custom output cache providers to change the way data are cached. For example, one of my customer wanted that some actions were cached on the file system to avoid loss of cache during an application pool reboot.
Be careful by using the following code because in many scenarios it can’t be used as it is
(in a web farm, for example).
A custom output cache provider is a simple class that inherits from OutputCacheProvider and overrides these methods :
- Add : adds an entry in the cache
- Get : gets an entry from the cache
- Remove : removes an entry from the cache
- Set : updates an entry in the cache
Cached items must be serializable. In this sample, the following class will be used :
[Serializable]
public class CachedItem
{
public object Item { get; set; }
public DateTime UtcExpiry { get; set; }
}
In order to save an item on the file system the two following methods will be used. The first allows to get a file path from the cache key (generated by asp.net) and the second allows to save a CachedItem on the disk :
private string GetFilePathFromKey(string key)
{
foreach (var invalidChar in Path.GetInvalidFileNameChars())
key = key.Replace(invalidChar, '_');
return Path.Combine(CacheDirectory, key);
}
private void SaveCachedItem(CachedItem cachedItem, string filePath)
{
if (File.Exists(filePath))
File.Delete(filePath);
using (var stream = File.OpenWrite(filePath))
{
var binaryFormatter = new BinaryFormatter();
binaryFormatter.Serialize(stream, cachedItem);
}
}
The Add method :
public override object Add(string key, object entry, DateTime utcExpiry)
{
string filePath = GetFilePathFromKey(key);
var cachedItem = GetCachedItem(filePath);
if (cachedItem != null && cachedItem.UtcExpiry.ToUniversalTime() <= DateTime.UtcNow)
{
Remove(key);
}
else if (cachedItem != null)
{
return cachedItem.Item;
}
SaveCachedItem(new CachedItem()
{
Item = entry,
UtcExpiry = utcExpiry
}, filePath);
return entry;
}
The Get method :
public override object Get(string key)
{
string filePath = GetFilePathFromKey(key);
var cachedItem = GetCachedItem(filePath);
if (cachedItem != null)
{
if (cachedItem.UtcExpiry.ToUniversalTime() <= DateTime.UtcNow)
{
Remove(key);
}
else
{
return cachedItem.Item;
}
}
return null;
}
The Remove method :
public override void Remove(string key)
{
string filePath = GetFilePathFromKey(key);
if (File.Exists(filePath))
File.Delete(filePath);
}
The Set Method :
public override void Set(string key, object entry, DateTime utcExpiry) {
string filePath = GetFilePathFromKey(key);
var cachedItem = new CachedItem() {Item = entry, UtcExpiry = utcExpiry};
SaveCachedItem(cachedItem, filePath);
}
Now that the output cache provider is created, it can be registered in the web.config file of the application, in the caching section :
<system.web>
<caching>
<outputCache>
<providers>
<add name="FileSystemOutputCacheProvider"
type="Samples.FileSystemOutputCacheProvider, Samples"/>
</providers>
</outputCache>
</caching>
The last step consists to override the GetOutputCacheProviderName method in the Global.asax file. Actually this method is called by the ASP.NET Framework when an OutputCache attribute (or an OutputCache directive) is used. The method returns the name of the output cache provider to use in the current context. For example, if you want to use the FileSystemOutputCacheProvider for the Details action of the Products controller, you can use this code :
public override string GetOutputCacheProviderName(HttpContext context)
{
if (context.Request.RawUrl.ToUpper().Contains("PRODUCTS/DETAILS")) {
return "FileSystemOutputCacheProvider";
}
return base.GetOutputCacheProviderName(context);
}
As mentioned previously you just have to set an OutputCache attribute on the action Details of the Products controller to use the custom output cache provider :
public class ProductsController : Controller
{
[OutputCache(Duration = 3600, VaryByParam = "id")]
public ActionResult Details(int id)
{
//long operation
Thread.Sleep(2000);
ViewBag.ProductId = id;
return View();
}
}
Download the source code here.
Hope this helps 
read more